About Me
Seasoned full stack developer with over 8 years of expertise crafting sophisticated web applications and bringing innovative tech products from concept to deployment. - My background in both tech and fitness helps me create solutions for people like me, busy professionals who want efficient tools that actually work.
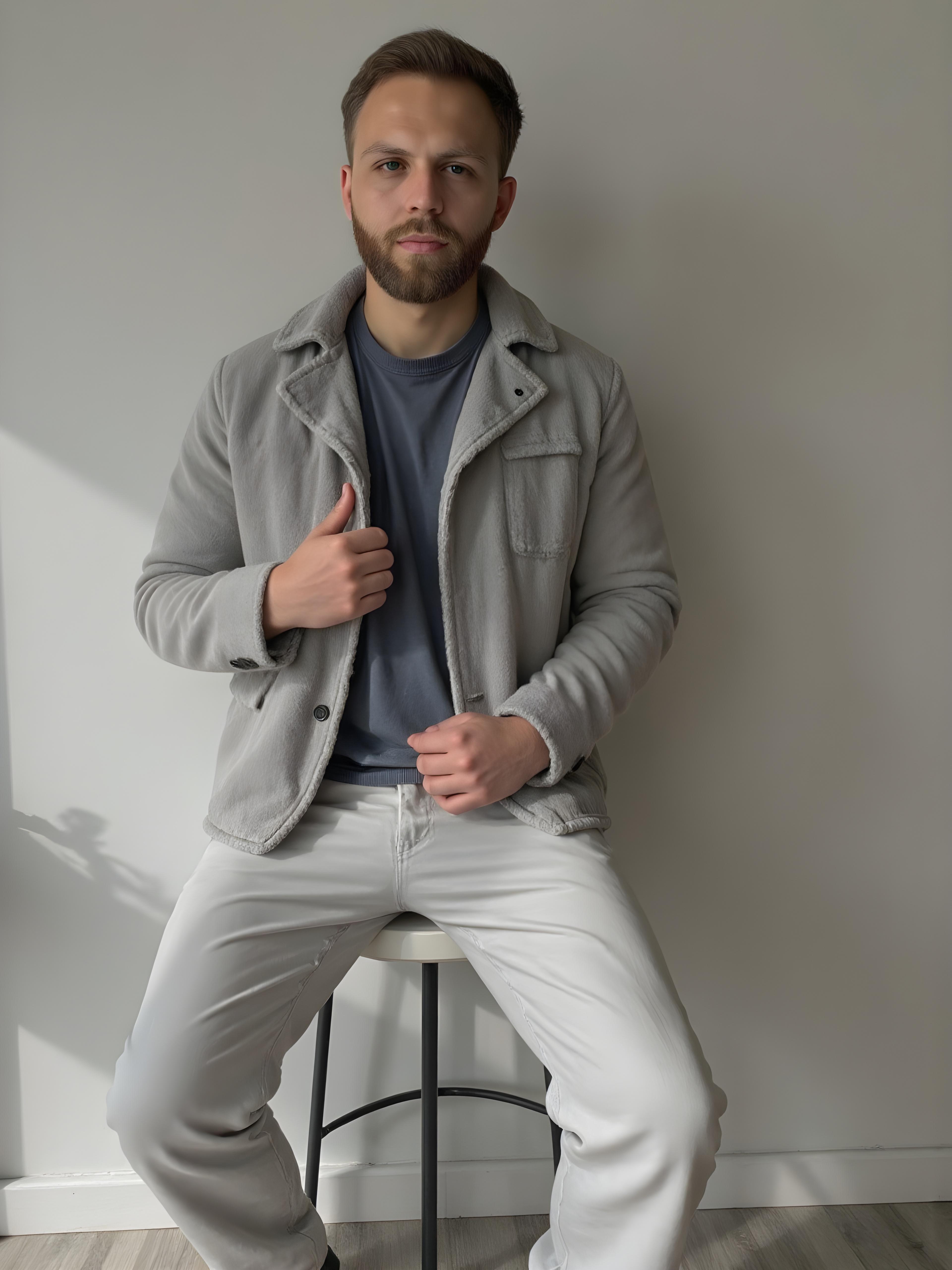
Projects
Experience
Senior Frontend Developer
ABN AMRO Bank N.V.
Jun 2024 - Present
Web Developer
FirstView - Digital Experience Agency
Jan 2021 - Mar 2023
Web Designer
D2D Digital Marketing
Apr 2015 - Jun 2017
UI Designer & Web Developer
Sportpro South Africa
Aug 2017 - Jan 2021
Frontend Developer
ShareValue B.V.
Apr 2023 - Mar 2024
Skills
Full Stack Web Development
UX Design
Framer Development
Product Development